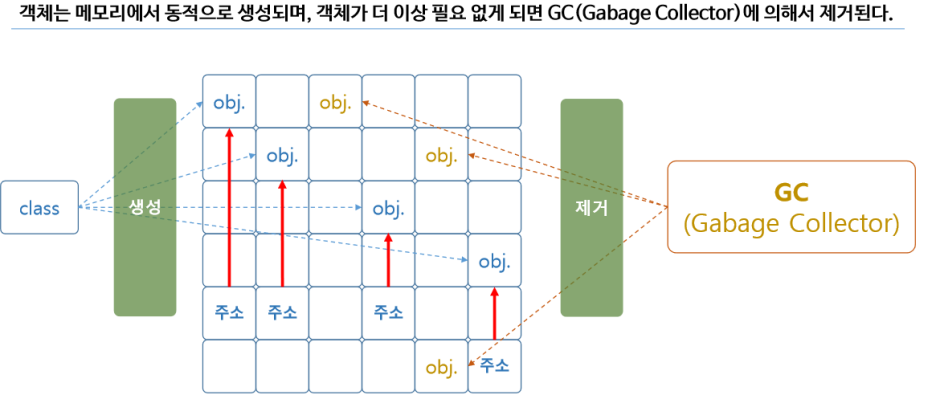
사진 설명을 입력하세요.
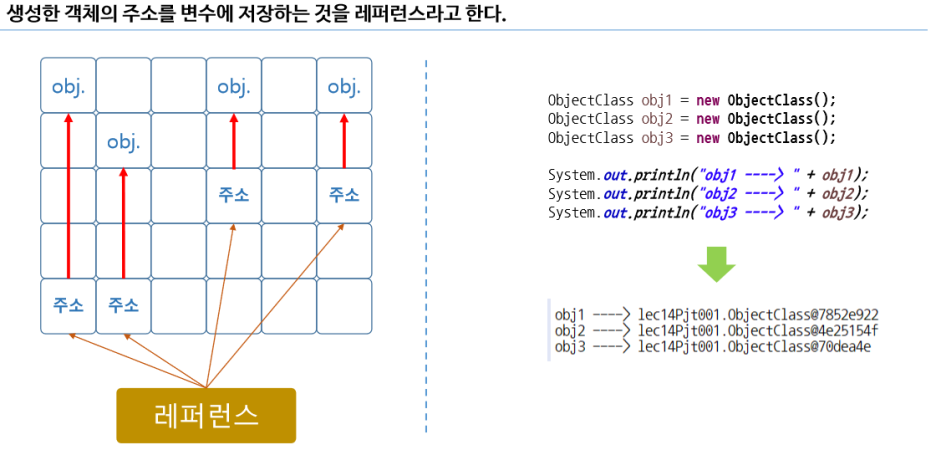
사진 설명을 입력하세요.
public class MainClass {
public static void main(String[] args) {
//생성한 객체의 주소를 변수에 저장하는것 --> 레퍼런스
ObjectClass obj1 = new ObjectClass();
ObjectClass obj2 = new ObjectClass();
ObjectClass obj3 = new ObjectClass();
System.out.println("obj1 ----> " + obj1);
System.out.println("obj2 ----> " + obj2);
System.out.println("obj3 ----> " + obj3);
/*결과값
* 클래스가 같아도 위치는 다른공간에존재한다. -> 다 다른 객체
* obj1 ----> ObjectClass@7852e922
* obj2 ----> ObjectClass@4e25154f
* obj3 ----> ObjectClass@70dea4e
*/
사진 설명을 입력하세요.
if(obj1 == obj2) { // obj1, obj2가 같은지 비교
System.out.println("obj1 == obj2"); //같으면 obj1 == obj2 출력
} else {
System.out.println("obj1 != obj2"); // 다르면 obj1 != obj2 출력
}
if(obj2 == obj3) {
System.out.println("obj2 == obj3");
} else {
System.out.println("obj2 != obj3");
}
if(obj1 == obj3) {
System.out.println("obj1 == obj3");
} else {
System.out.println("obj1 != obj3");
}
/*결과값
* obj1 != obj2
* obj2 != obj3
* obj1 != obj3
*/
사진 설명을 입력하세요.
System.out.println("obj1 ----> " + obj1);
obj1.getInfo();
//레퍼런스에 null이 저장되면 객제의 연결이 끊기며, 더이상 객체를 이용할 수 없다.
obj1 = null; // 레퍼런스 X
System.out.println("obj1 ---->" + obj1);
obj1.getInfo();
/*결과값
* obj1 ----> ObjectClass@7852e922
* == getIndo() --
* obj1 ---->null
* Exception in thread "main" java.lang.NullPointerException
* at MainClass.main(MainClass.java:49)
*/
//레퍼런스(가리킨다)
//객체는 메모리에서 동적으로 생성되며, 객체가 더이상 필요 없게 되면 GC(Gabage Collector)에 의해 제거된다.
//MainClass.java
public class MainClass {
public static void main(String[] args) {
//생성한 객체의 주소를 변수에 저장하는것 --> 레퍼런스
ObjectClass obj1 = new ObjectClass();
ObjectClass obj2 = new ObjectClass();
ObjectClass obj3 = new ObjectClass();
System.out.println("obj1 ----> " + obj1);
System.out.println("obj2 ----> " + obj2);
System.out.println("obj3 ----> " + obj3);
/*결과값
* 클래스가 같아도 위치는 다른공간에존재한다. -> 다 다른 객체
* obj1 ----> ObjectClass@7852e922
* obj2 ----> ObjectClass@4e25154f
* obj3 ----> ObjectClass@70dea4e
*/
if(obj1 == obj2) { // obj1, obj2가 같은지 비교
System.out.println("obj1 == obj2"); //같으면 obj1 == obj2 출력
} else {
System.out.println("obj1 != obj2"); // 다르면 obj1 != obj2 출력
}
if(obj2 == obj3) {
System.out.println("obj2 == obj3");
} else {
System.out.println("obj2 != obj3");
}
if(obj1 == obj3) {
System.out.println("obj1 == obj3");
} else {
System.out.println("obj1 != obj3");
}
/*결과값
* obj1 != obj2
* obj2 != obj3
* obj1 != obj3
*/
System.out.println("obj1 ----> " + obj1);
obj1.getInfo();
//레퍼런스에 null이 저장되면 객제의 연결이 끊기며, 더이상 객체를 이용할 수 없다.
obj1 = null; // 레퍼런스 X
System.out.println("obj1 ---->" + obj1);
obj1.getInfo();
/*결과값
* obj1 ----> ObjectClass@7852e922
* == getIndo() --
* obj1 ---->null
* Exception in thread "main" java.lang.NullPointerException
* at MainClass.main(MainClass.java:49)
*/
}
}
//ObjectClass.java
public class ObjectClass {
public void getInfo() {
System.out.println(" == getIndo() -- ");
}
}
결과값
obj1 ----> ObjectClass@7852e922
obj2 ----> ObjectClass@4e25154f
obj3 ----> ObjectClass@70dea4e
obj1 != obj2
obj2 != obj3
obj1 != obj3
obj1 ----> ObjectClass@7852e922
== getIndo() --
obj1 ---->null
Exception in thread "main" java.lang.NullPointerException
at MainClass.main(MainClass.java:49)
'Develop > JAVA' 카테고리의 다른 글
JAVA 객체 패키지와 static (0) | 2023.10.21 |
---|---|
JAVA 객체 생성자 소멸자 this키워드 (0) | 2023.10.21 |
JAVA 메서드(선언, 호출, 중복) (0) | 2023.10.21 |
JAVA 객체지향 프로그래밍 (0) | 2023.10.21 |
JAVA Collections(List,Map) (0) | 2023.10.17 |